User management
Handling users is important for most types of applications, and certainly so for interactive, conversational applications. Narratory make it easy to authenticate users and create different flows for new and returning users as described on this page.
#
Authenticating usersIn most applications, you want to authenticate users since you simply need to know who they are in order to provide a personalized experience. This typically is done differently on different platforms.
Narratory currently, out of the box, supports authenticating users for Google Assistant/Google Actions. The only thing you need to do is to add a BotTurn with answers including the built-in intents SigninSuccess
and SigninFailed
AND importantly to enable "Google Sign in" Account linking on the Google Assistant console. Then Narratory will do the rest for you.
Enabling Account linking in the Google Assistant console:
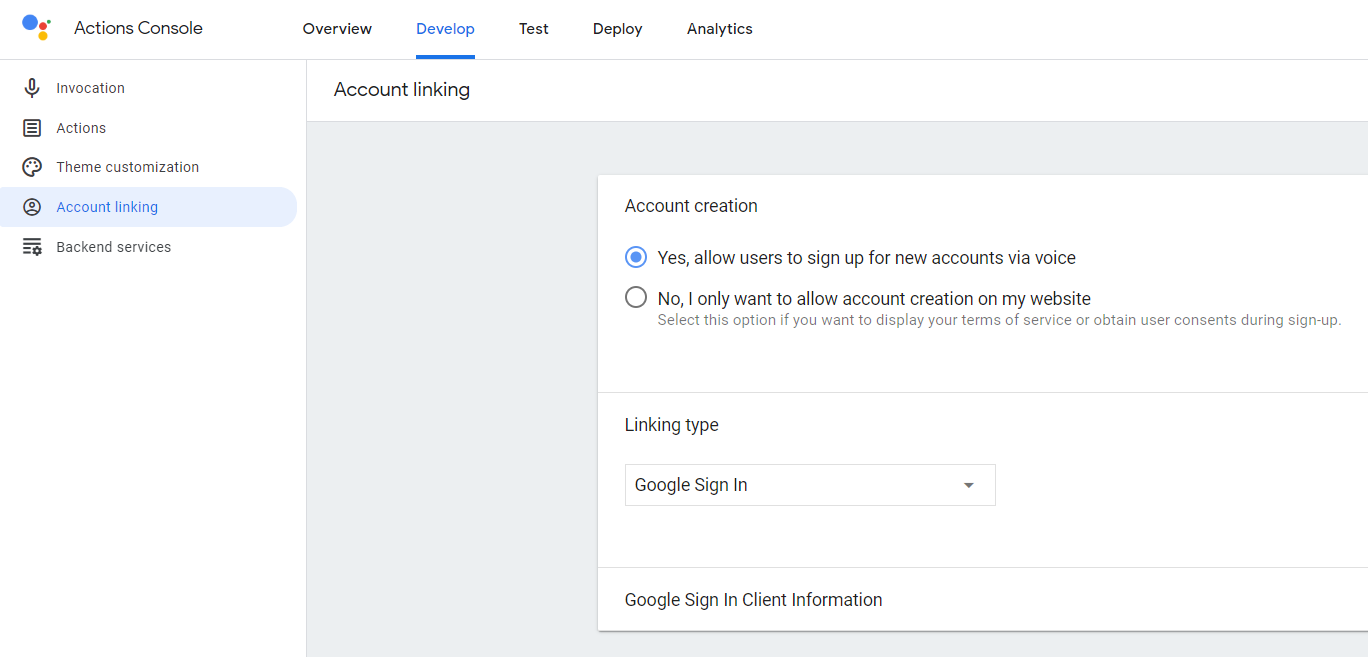
#
Signing inImportant note: Google offers a standard flow for asking users to share their information with voice apps. The only addition to this flow you have to do is to add a phrase that assistant will say before their automatic phrases begin. It basically means filling in the gap here: "____, you’ll need an account with my test app. To create a new one for you, I’ll just need some info. If you want more details, say "Tell me more." So, can I ask Google for your name, email address, and profile picture?". See the script below for an example with some context.
Support for authentication on other platforms will be added shortly.
import { BotTurn, SignInSuccess, SignInFailed } from "narratory"
const beforeAuth = "Welcome to my dinner reservation app!"
const auth: BotTurn = { say: "To make reservations", user: [ { intent: SignInSuccess, bot: "Now, let's proceed." }, { intent: SignInFailed, bot: { say: "Unfortunately you have to sign in for me to be able to help you", goto: "END" }} ]}
const booking: BotTurn = { say: "What can I help you with?" // .... other things would happen here..}
const end: BotTurn = { say: "Goodbye!", label: "END"}
const narrative = [beforeAuth, auth, booking, end]
The above snippet shows how to build a sign-in step for Google Assistant app. If you try this on different platforms, you will get a custom error message.
The user-experience offered by the above is visualized by the following script for a happy path:
Bot: Welcome to my dinner reservation app!Bot: To make reservations, you’ll need an account with my test app. To create a new one for you, I’ll just need some info. If you want more details, say "Tell me more." So, can I ask Google for your name, email address, and profile picture?User: YesBot: OK. By the way, our Terms of Service and our Privacy Policy are on our website. You can look them over at any time. Now, ready to make your new my test app account?User: YesBot: Great, your new my test app account is set up. You'll get a confirmation email soon.Bot: Now, let's proceedBot: What can I help you with?...
If the user says no to Googles automatic questions, the following flow will happen instead:
Bot: Welcome to my dinner reservation app!Bot: To make reservations, you’ll need an account with my test app. To create a new one for you, I’ll just need some info. If you want more details, say "Tell me more." So, can I ask Google for your name, email address, and profile picture?User: NoBot: OK. Just so you know, that means you won't be able to use your account with my test app. If you change your mind, you can always come back and sign in then.Bot: Unfortunately you have to sign in for me to be able to help youBot: Goodbye
#
Returning usersReturning users will automatically be signed in on any other device where they are logged in with the same Google account. Thus, the whole auth BotTurn as shown in the previous section will be ignored and the user-experience for returning users will instead be:
Bot: Welcome to my dinner reservation app!Bot: What can I help you with?...
Typically you want to handle returning visitors in a different way than new users, see the next section for how to do this.
#
Handling guests/verified users and new/returning visitorsYou typically want to add greet returning users in a different way than new users, and in addition you might not be capable of giving full service to guest users (in the Google Assistant ecosystem a guest user is a user not logged in with their Google account). System-set variables exist for these cases that you can use in conditionals and API-calls.
Note: These variables are currently only supported for Google Assistant
An example showing how to use these variables is shown below:
const welcomeNew: BotTurn = { cond: { user_returning: false }, say: "Hi! I think you are new here!",}
const welcomeReturning: BotTurn = { cond: { user_returning: true }, say: "Welcome back!"}
const guestDenial: BotTurn = { cond: { user_guest: true }, say: "Unfortunately I can only serve logged-in users", goto: "END"}
const end : BotTurn = { label: "END", say: "Goodbye!"}
const narrative = [welcomeNew, welcomeReturning, guestDenial, end]